Create a new ASP.NET MVC project named XMLToHTML.
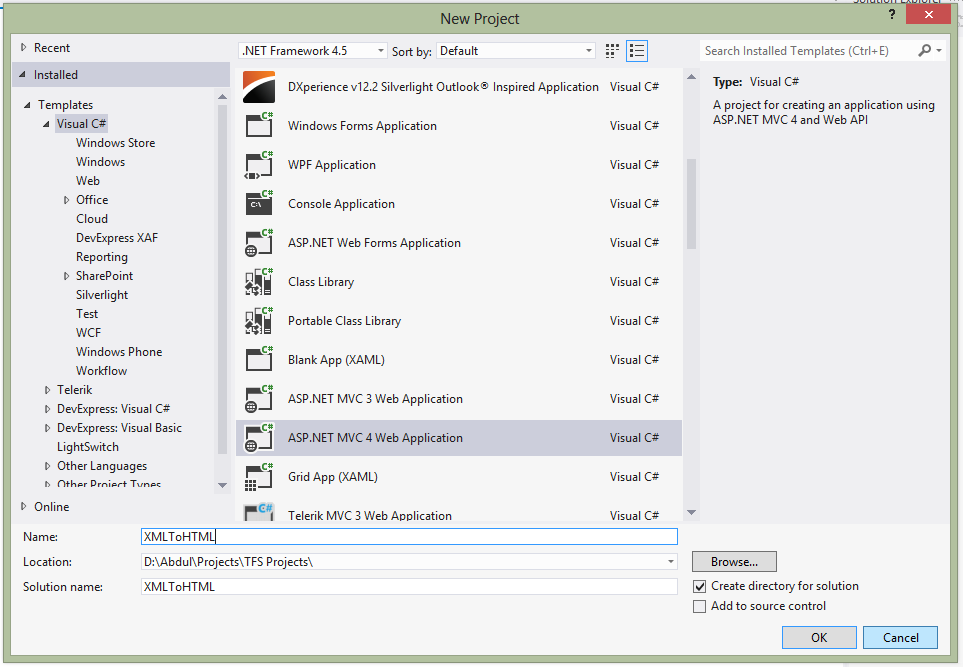
Select Empty Template.
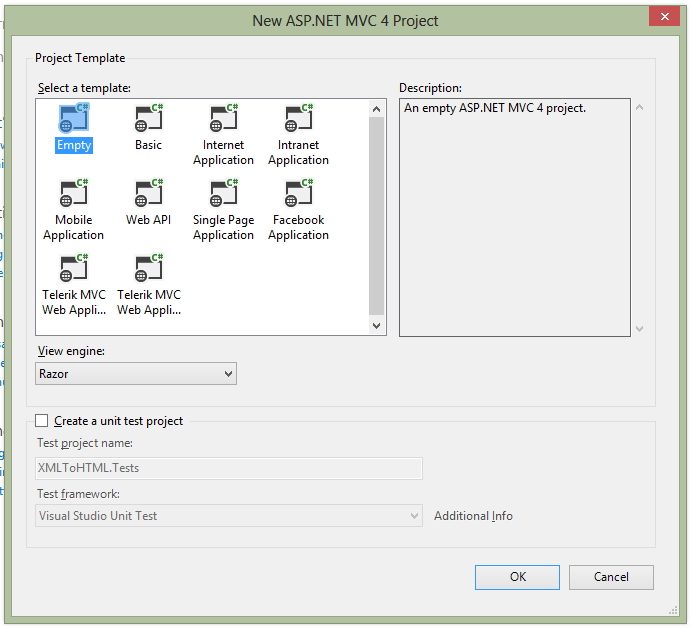
Add a xml file Employees.xml with below markup:
Add a xslt file Employees.xslt with below markup:
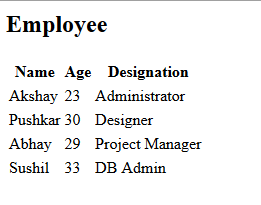
Select Empty Template.
Add a xml file Employees.xml with below markup:
<?xml version="1.0" encoding="utf-8" ?> <Employees> <Employee name="Akshay" age="23" designation="Administrator"></Employee> <Employee name="Pushkar" age="30" designation="Designer"></Employee> <Employee name="Abhay" age="29" designation="Project Manager"></Employee> <Employee name="Sushil" age="33" designation="DB Admin"></Employee> </Employees>
Add a xslt file Employees.xslt with below markup:
<?xml version="1.0" encoding="utf-8"?> <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl"> <xsl:template match="/*"> <table> <tr> <th>Name</th> <th>Age</th> <th>Designation</th> </tr> <xsl:for-each select="Employee"> <tr> <td> <xsl:value-of select="@name"></xsl:value-of> </td> <td> <xsl:value-of select="@age"></xsl:value-of> </td> <td> <xsl:value-of select="@designation"></xsl:value-of> </td> </tr> </xsl:for-each> </table> </xsl:template> </xsl:stylesheet>Add a new controller EmployeesController with below code:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace XMLToHTML.Controllers { public class EmployeesController : Controller { public ActionResult Index() { string employeesXML = System.IO.File.ReadAllText(Server.MapPath("XML/Employees.xml")); ViewBag.EmployeesXML = employeesXML; return View(); } } }Add a HtmlHelperExtensions class with below code:
using System; using System.Collections.Generic; using System.IO; using System.Linq; using System.Web; using System.Web.Mvc; using System.Xml; using System.Xml.Xsl; namespace XMLToHTML.Helper { public static class HtmlHelperExtensions { /// /// Applies an XSL transformation to an XML document. /// public static HtmlString RenderXml(this HtmlHelper helper, string xml, string xsltPath) { XsltArgumentList args = new XsltArgumentList(); XslCompiledTransform t = new XslCompiledTransform(); t.Load(xsltPath); XmlReaderSettings settings = new XmlReaderSettings(); settings.DtdProcessing = DtdProcessing.Parse; settings.ValidationType = ValidationType.DTD; using (XmlReader reader = XmlReader.Create(new StringReader(xml), settings)) { StringWriter writer = new StringWriter(); t.Transform(reader, args, writer); HtmlString htmlString = new HtmlString(writer.ToString()); return htmlString; } } } }Add a view for Employees Index action with below marup.
@using XMLToHTML.Helper; @{ ViewBag.Title = "Index"; } <h2>Employee</h2> @Html.RenderXml(ViewBag.EmployeesXML as string, Server.MapPath("~/XSLT/Employees.xslt"))Build and browse the project. You should see the rendered html.
comments powered by
Disqus